Spring中JdbcTemplate的使用
JdbcTemplate 概述
它是 spring 框架中提供的一个对象,是对原始 Jdbc API 对象的简单封装。spring 框架为我们提供了很多的操作模板类。
- 操作关系型数据的:
- JdbcTemplate
- HibernateTemplate
- 操作 nosql 数据库的:
- 操作消息队列的:
我们今天的主角在 spring-jdbc-5.0.2.RELEASE.jar
中,我们在导包的时候,除了要导入这个 jar 包外,还需要导入一个 spring-tx-5.0.2.RELEASE.jar
(它是和事务相关的)。
配置数据源
配置 C3P0 数据源
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"> <property name="driverClass" value="com.mysql.jdbc.Driver"></property> <property name="jdbcUrl" value="jdbc:mysql:///spring_day02"></property> <property name="user" value="root"></property> <property name="password" value="1234"></property> </bean> </beans>
|
配置 DBCP 数据源
1 2 3 4 5 6 7 8 9 10 11 12 13
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"></property> <property name="url" value="jdbc:mysql:// /spring_day02"></property> <property name="username" value="root"></property> <property name="password" value="1234"></property> </bean> </beans>
|
配置 spring 内置数据源
spring 框架也提供了一个内置数据源,我们也可以使用 spring 的内置数据源,它就在
spring-jdbc-5.0.2.REEASE.jar 包中
1 2 3 4 5 6 7 8 9 10 11 12 13
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"></property> <property name="url" value="jdbc:mysql:///spring_day02"></property> <property name="username" value="root"></property> <property name="password" value="1234"></property> </bean> </beans>
|
将数据库连接的信息配置到属性文件中
【定义属性文件】
1 2 3 4
| jdbc.driverClass=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql:///spring_day02 jdbc.username=root jdbc.password=123
|
【引入外部的属性文件】
- 一种方式:
1 2 3 4
| <bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"> <property name="location" value="classpath:jdbc.properties"/> </bean>
|
- 另一种方式:
1
| <context:property-placeholder location="classpath:jdbc.properties"/>
|
然后我们就可以配置Spring的IOC来使用jdbcTemplate
- 问题:当我们有很多个dao时,每个dao都有一些重复性代码
1 2 3 4 5
| private JdbcTemplate jdbcTemplate; public void setJdbcTemplate(JdbcTemplate jdbcTemplate) { this.jdbcTemplate = jdbcTemplate; }
|
- 解决方式一:手动创建一个JdbcDaoSupport对象,这个对象里面放重复代码,接着让dao继承它
- 解决方式二:使用Spring自带的JdbcDaoSupport对象,它的源码和手动创建的同理
两者的区别:
- 解决方式一适用于所有配置方式
- 解决方式二因为不能修改Spring源码文件所以只能用xml配置文件的方式,不能使用注解
Spring中的事务控制
Spring 事务控制我们要明确的
- 第一:JavaEE 体系进行分层开发,事务处理位于业务层,Spring 提供了分层设计业务层的事务处理解决方案。
- 第二:spring 框架为我们提供了一组事务控制的接口。这组接口是在spring-tx-5.0.2.RELEASE.jar 中。
- 第三:spring 的事务控制都是基于 AOP 的,它既可以使用编程的方式实现,也可以使用配置的方式实现。我们学习的重点是使用配置的方式实现。
Spring 中事务控制的 API 介绍
此接口是 spring 的事务管理器,它里面提供了我们常用的操作事务的方法,我们在开发中都是使用它的实现类
真正管理事务的对象:
- org.springframework.jdbc.datasource.DataSourceTransactionManager 使用 SpringJDBC 或 iBatis 进行持久化数据时使用
- org.springframework.orm.hibernate5.HibernateTransactionManager 使用Hibernate 版本进行持久化数据时使用
TransactionDefinition
它是事务的定义信息对象,里面有如下方法
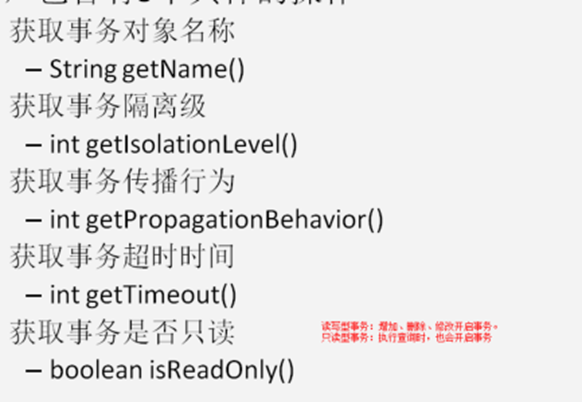
事务的隔离级别
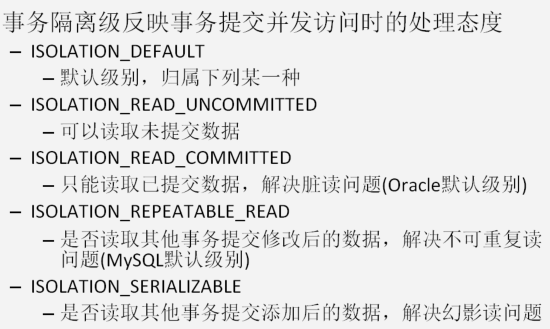
事务的传播行为
- REQUIRED:如果当前没有事务,就新建一个事务,如果已经存在一个事务中,加入到这个事务中。一般的选择(默认值)
- SUPPORTS:支持当前事务,如果当前没有事务,就以非事务方式执行(没有事务)
- MANDATORY:使用当前的事务,如果当前没有事务,就抛出异常
- REQUERS_NEW:新建事务,如果当前在事务中,把当前事务挂起。
- NOT_SUPPORTED:以非事务方式执行操作,如果当前存在事务,就把当前事务挂起
- NEVER:以非事务方式运行,如果当前存在事务,抛出异常
- NESTED:如果当前存在事务,则在嵌套事务内执行。如果当前没有事务,则执行 REQUIRED 类似的操作。
TransactionStatus
此接口提供的是事务具体的运行状态,方法介绍如下图:
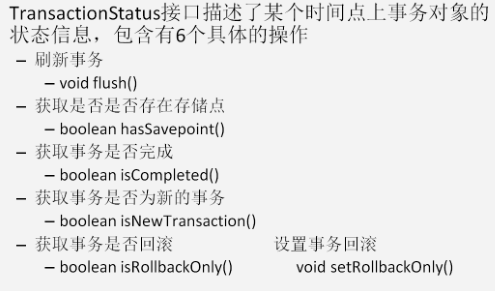
基于 XML 的声明式事务控制(配置方式)重点
导入约束
此处需要导入 aop 和 tx 两个名称空间
1 2 3 4 5 6 7 8 9 10 11 12
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> </beans>
|
Spring配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="accountService" class="com.itheima.service.impl.AccountServiceImpl"> <property name="accountDao" ref="accountDao"></property> </bean>
<bean id="accountDao" class="com.itheima.dao.impl.AccountDaoImpl"> <property name="dataSource" ref="dataSource"></property> </bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"></property> <property name="url" value="jdbc:mysql://localhost:3306/eesy"></property> <property name="username" value="root"></property> <property name="password" value="1234"></property> </bean>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"></property> </bean>
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes> <tx:method name="*" propagation="REQUIRED" read-only="false"/> <tx:method name="find*" propagation="SUPPORTS" read-only="true"></tx:method> </tx:attributes> </tx:advice>
<aop:config> <aop:pointcut id="pt1" expression="execution(* com.itheima.service.impl.*.*(..))"></aop:pointcut> <aop:advisor advice-ref="txAdvice" pointcut-ref="pt1"></aop:advisor> </aop:config>
</beans>
|
基于注解的配置方式
配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<context:component-scan base-package="com.itheima"></context:component-scan> <bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate"> <property name="dataSource" ref="dataSource"></property> </bean> <bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"></property> <property name="url" value="jdbc:mysql://localhost:3306/spring_day02"></property> <property name="username" value="root"></property> <property name="password" value="1234"></property> </bean> <bean id="transactionManager"class="org.springframework.jdbc.datasource.DataSourceTransactionManager"> <property name="dataSource" ref="dataSource"></property> </bean>
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
|
注解配置
在业务层使用@Transactional 注解
1
| @Transactional(readOnly=true,propagation=Propagation.SUPPORTS)
|
该注解的属性和 xml 中的属性含义一致。该注解可以出现在接口上,类上和方法上。
- 出现接口上,表示该接口的所有实现类都有事务支持。
- 出现在类上,表示类中所有方法有事务支持
- 出现在方法上,表示方法有事务支持。
以上三个位置的优先级:方法 > 类 > 接口
不使用 xml 的配置方式
1 2 3 4 5
| @Configuration @EnableTransactionManagement public class SpringTxConfiguration {
}
|
Spring5 的新特性[了解]
jdk 版本要求
spring5.0 在 2017 年 9 月发布了它的 GA(通用)版本。该版本是基于 jdk8 编写的,所以 jdk8 以下版本将无法使用。同时,可以兼容 jdk9 版本。
tomcat 版本要求 8.5 及以上。
注:我们使用 jdk8 构建工程,可以降版编译。但是不能使用 jdk8 以下版本构建工程。
由于 jdk 和 tomcat 版本的更新,我们的 IDE 也需要同时更新。
Spring利用了 jdk8 版本更新的内容
第一:基于 JDK8 的反射增强,jdk1.8比jdk1.7反射创建对象快了不止100倍
###第二:@NonNull 注解和@Nullable 注解的使用
用 @Nullable 和 @NotNull 注解来显示表明可为空的参数和以及返回值。这样就够在编译的时候处
理空值而不是在运行时抛出 NullPointerExceptions。
###第三:日志记录方面
Spring Framework 5.0 带来了 Commons Logging 桥接模块的封装, 它被叫做 spring-jcl 而
不是标准的 Commons Logging。当然,无需任何额外的桥接,新版本也会对 Log4j 2.x, SLF4J, JUL
( java.util.logging) 进行自动检测。
核心容器的更新
Spring Framework 5.0 现在支持候选组件索引作为类路径扫描的替代方案。该功能已经在类路径扫描器中
添加,以简化添加候选组件标识的步骤。
应用程序构建任务可以定义当前项目自己的 META-INF/spring.components 文件。在编译时,源模型是
自包含的,JPA 实体和 Spring 组件是已被标记的。
从索引读取实体而不是扫描类路径对于小于 200 个类的小型项目是没有明显差异。但对大型项目影响较大。
加载组件索引开销更低。因此,随着类数的增加,索引读取的启动时间将保持不变。
加载组件索引的耗费是廉价的。因此当类的数量不断增长,加上构建索引的启动时间仍然可以维持一个常数,
不过对于组件扫描而言,启动时间则会有明显的增长。
这个对于我们处于大型 Spring 项目的开发者所意味着的,是应用程序的启动时间将被大大缩减。虽然 20
或者 30 秒钟看似没什么,但如果每天要这样登上好几百次,加起来就够你受的了。使用了组件索引的话,就能帮
助你每天过的更加高效。
JetBrains Kotlin 语言支持
Kolin概述:是一种支持函数式编程编程风格的面向对象语言。Kotlin 运行在 JVM 之上,但运行环境并不
限于 JVM。
Kolin 的示例代码:
1 2 3 4 5 6 7 8 9 10 11
| { ("/movie" and accept(TEXT_HTML)).nest { GET("/", movieHandler::findAllView) GET("/{card}", movieHandler::findOneView) } ("/api/movie" and accept(APPLICATION_JSON)).nest { GET("/", movieApiHandler::findAll) GET("/{id}", movieApiHandler::findOne) } }
|
Kolin 注册 bean 对象到 spring 容器:
1 2 3 4
| val context = GenericApplicationContext { registerBean() registerBean { Cinema(it.getBean()) } }
|
响应式编程风格
此次 Spring 发行版本的一个激动人心的特性就是新的响应式堆栈 WEB 框架。这个堆栈完全的响应式且非
阻塞,适合于事件循环风格的处理,可以进行少量线程的扩展。
Reactive Streams 是来自于 Netflix, Pivotal, Typesafe, Red Hat, Oracle, Twitter 以及
Spray.io 的工程师特地开发的一个 API。它为响应式编程实现的实现提供一个公共的 API,好实现
Hibernate 的 JPA。这里 JPA 就是这个 API, 而 Hibernate 就是实现。
Reactive Streams API 是 Java 9 的官方版本的一部分。在 Java 8 中, 你会需要专门引入依赖来使
用 Reactive Streams API。
Spring Framework 5.0 对于流式处理的支持依赖于 Project Reactor 来构建, 其专门实现了
Reactive Streams API。
Spring Framework 5.0 拥有一个新的 spring-webflux 模块,支持响应式 HTTP 和 WebSocket 客
户端。Spring Framework 5.0 还提供了对于运行于服务器之上,包含了 REST, HTML, 以及 WebSocket 风
格交互的响应式网页应用程序的支持。
在 spring-webflux 中包含了两种独立的服务端编程模型:
基于注解:使用到了@Controller 以及 Spring MVC 的其它一些注解;
使用 Java 8 lambda 表达式的函数式风格的路由和处理。
有 了 Spring Webflux, 你现在可以创建出 WebClient, 它是响应式且非阻塞的,可以作为
RestTemplate 的一个替代方案。
这里有一个使用 Spring 5.0 的 REST 端点的 WebClient 实现:
1 2 3 4 5 6
| WebClient webClient = WebClient.create() Mono person = webClient.get() .uri("http://localhost:8080/movie/42") .accept(MediaType.APPLICATION_JSON) .exchange() .then(response -> response.bodyToMono(Movie.class))
|
Junit5 支持
完全支持 JUnit 5 Jupiter,所以可以使用 JUnit 5 来编写测试以及扩展。此外还提供了一个编程以及
扩展模型,Jupiter 子项目提供了一个测试引擎来在 Spring 上运行基于 Jupiter 的测试。
另外,Spring Framework 5 还提供了在 Spring TestContext Framework 中进行并行测试的扩展。
针对响应式编程模型, spring-test 现在还引入了支持 Spring WebFlux 的 WebTestClient 集成测
试的支持,类似于 MockMvc,并不需要一个运行着的服务端。使用一个模拟的请求或者响应, WebTestClient
就可以直接绑定到 WebFlux 服务端设施。
你可以在这里找到这个激动人心的 TestContext 框架所带来的增强功能的完整列表。
当然, Spring Framework 5.0 仍然支持我们的老朋友 JUnit! 在我写这篇文章的时候, JUnit 5 还
只是发展到了 GA 版本。对于 JUnit4, Spring Framework 在未来还是要支持一段时间的。
依赖类库的更新
- 终止支持的类库
- Portlet.
- Velocity.
- JasperReports.
- XMLBeans.
- JDO.
- Guava.
- 支持的类库
- Jackson 2.6+
- EhCache 2.10+ / 3.0 GA
- Hibernate 5.0+
- JDBC 4.0+
- XmlUnit 2.x+
- OkHttp 3.x+
- Netty 4.1+