Swagger2快速入门
Swagger 是一个规范和完整的框架,用于生成、描述、调用和可视化 RESTful 风格的 Web 服务。总体目标是使客户端和文件系统作为服务器以同样的速度来更新。
官网:https://swagger.io/
构建spring-web或者springboot-web项目:
测试接口:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| @RestController @RequestMapping("/user") public class UserController {
@GetMapping("/{id}") public User getUser(@PathVariable("id") Integer id){ System.out.println(id); User user = new User(); user.setId(id); user.setName("name-"+id); return user; }
@DeleteMapping("/{id}") public boolean deleteUser(@PathVariable("id") Integer id){ System.out.println(id); return true; }
@PostMapping("/addUser") public boolean insertUser(@RequestBody User user){ System.out.println(user); System.out.println(user); return true; }
@PutMapping("/updateUser") public boolean updatetUser(@RequestBody User user){ System.out.println(user); System.out.println(user); return true; }
@GetMapping("/all") public List<User> findAll(){ User u1 = new User(); u1.setName("111"); u1.setId(1); User u2 = new User(); u2.setName("222"); u2.setId(2); List<User> users = Arrays.asList(u1, u2); System.out.println(users); return users; }
}
|
接口随便定义;
1.配置
1.1.项目引入依赖
工程pom.xml引入依赖:
1 2 3 4 5 6 7 8 9 10 11 12
| <dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.5.0</version> </dependency>
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.5.0</version> </dependency>
|
1.2 配置swagger
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| package com.swagger.config;
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import springfox.documentation.builders.ApiInfoBuilder; import springfox.documentation.builders.PathSelectors; import springfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket; import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration @EnableSwagger2 public class SwaggerApp {
@Bean public Docket createRestApi(){ return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() .apis(RequestHandlerSelectors.basePackage("com.swagger.controller")) .paths(PathSelectors.any()) .build(); }
private ApiInfo apiInfo() { return new ApiInfoBuilder() .title("学习Swagger标题") .description("用于swagger学习") .contact(new Contact("swagger-study","http://localhost:8080","xx@163.com")) .version("1.0.0") .build(); } }
|
1.3 访问swagger
1
| http://localhost:8080/swagger-ui.html
|
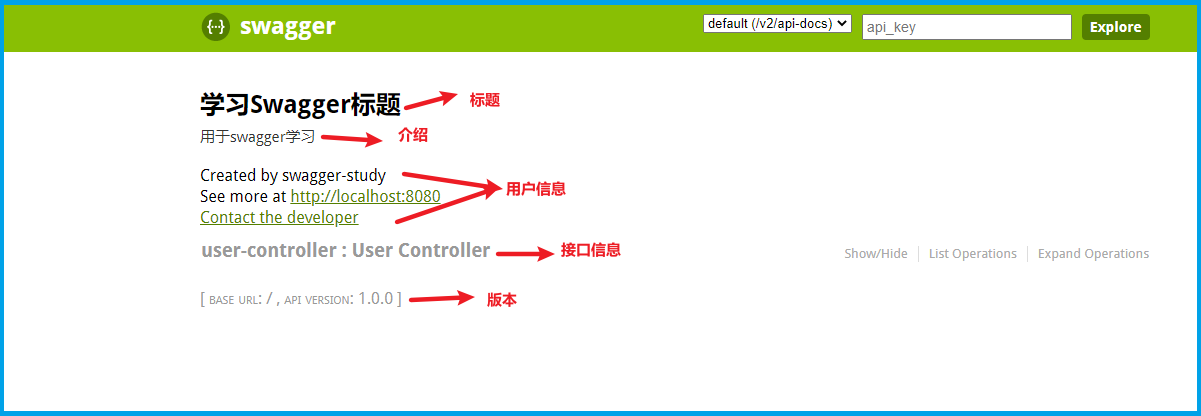
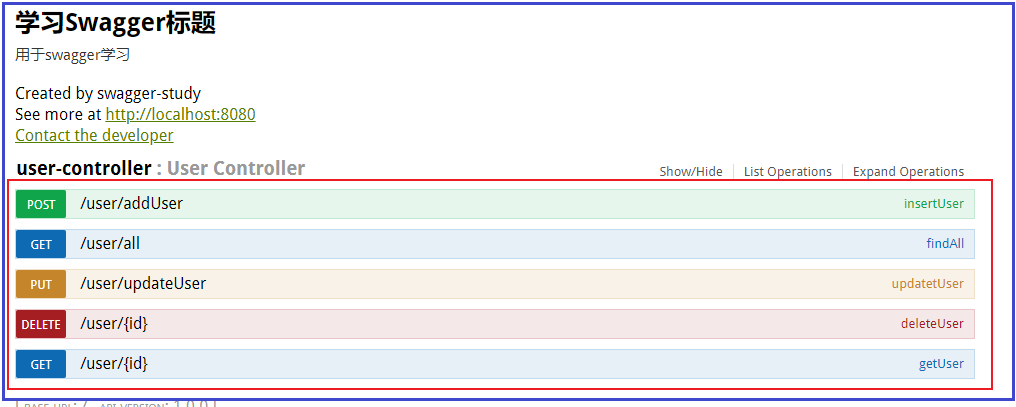
进入接口方法,可进行测试;
2. Swagger核心注解介绍
在默认配置下,apiweb界面不够丰富,可通过注解丰富api文档展示;
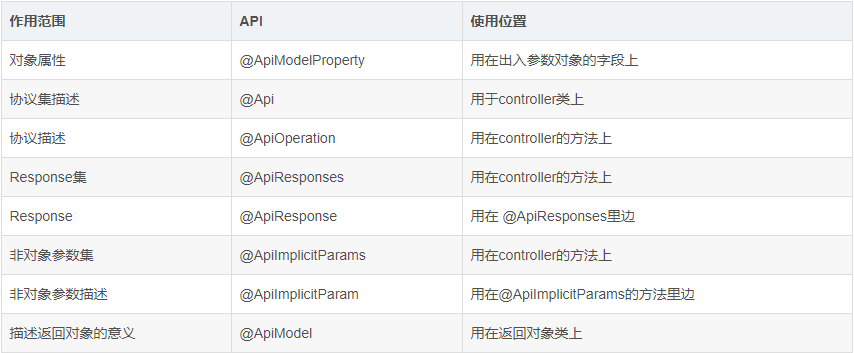
示例代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83
| package com.swagger.controller;
import com.swagger.vo.User; import io.swagger.annotations.*; import org.springframework.web.bind.annotation.*; import java.util.Arrays; import java.util.List;
@Api(tags = "访问接口") @RestController @RequestMapping("/user") public class UserController { @ApiOperation(value = "根据id查询用户信息") @ApiImplicitParam(name = "id",value = "用户ID",required = true,dataType = "Integer") @GetMapping("/{id}") public User getUser(@PathVariable("id") Integer id){ System.out.println(id); User user = new User(); user.setId(id); user.setName("name-"+id); return user; }
@DeleteMapping("/{id}") public boolean deleteUser(@PathVariable("id") Integer id){ System.out.println(id); return true; }
@ApiOperation(value = "添加用户信息",notes = "post请求,传入json格式user信息") @PostMapping("/addUser") public boolean insertUser(@RequestBody User user){ System.out.println(user); System.out.println(user); return true; } @ApiOperation(value = "更新用户信息",notes = "put请求,传入json格式user信息") @ApiImplicitParam(name = "user",value = "用户信息") @ApiResponse(response = Boolean.class,code = 200,message = "响应布尔值") @PutMapping("/updateUser") public boolean updatetUser(@RequestBody User user){ System.out.println(user); System.out.println(user); return true; }
@ApiOperation(value = "查询所有用户信息") @GetMapping("/all") public List<User> findAll(){ User u1 = new User(); u1.setName("111"); u1.setId(1); User u2 = new User(); u2.setName("222"); u2.setId(2); List<User> users = Arrays.asList(u1, u2); System.out.println(users); return users; }
@ApiOperation(value = "根据输入name和id创建用户信息") @ApiImplicitParams(value = { @ApiImplicitParam(name = "name",value = "姓名"), @ApiImplicitParam(name = "id",value = "编号") }) @GetMapping("/{name}/{id}") public User insertUser(@PathVariable("name")String name,@PathVariable("id")Integer id){ User user = new User(); user.setId(id); user.setName(name); System.out.println(user); return user; } }
|